We are going to connect to Dynamics 365 api , get a list of contacts using Web Api using a token
First thing we need to do is, resister an app in azure active directory
Go to portal.azure.com >Azure Active Directory > App Registrations > New application registration
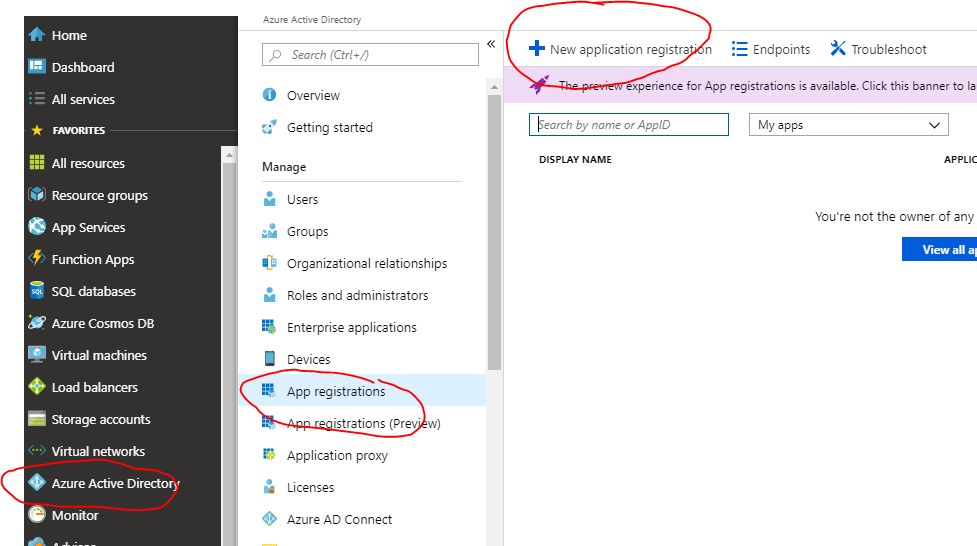
Give it a name and click on create button
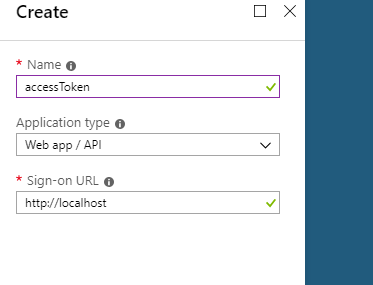
Then go to API permissions and select an API (in this case Dynamics CRM
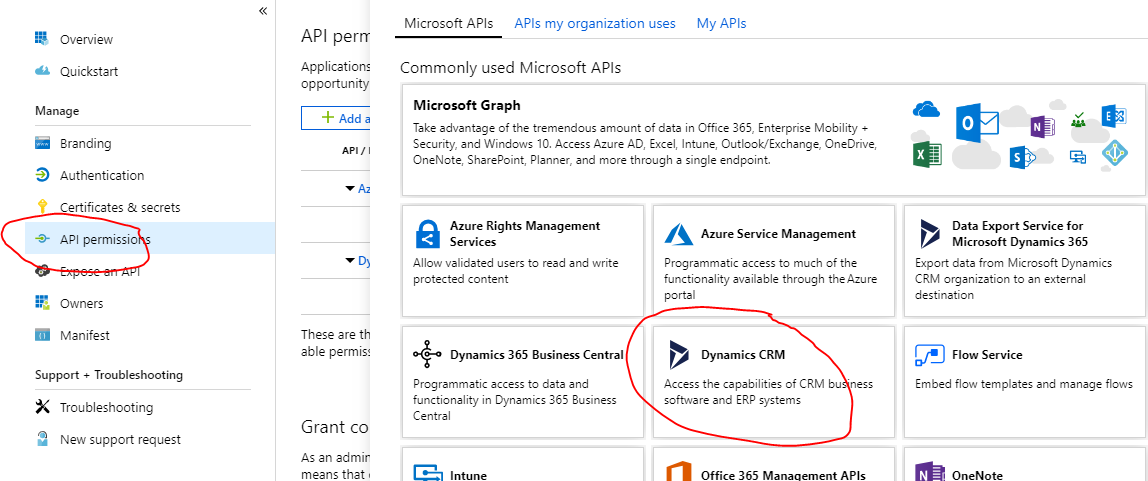
Select as below
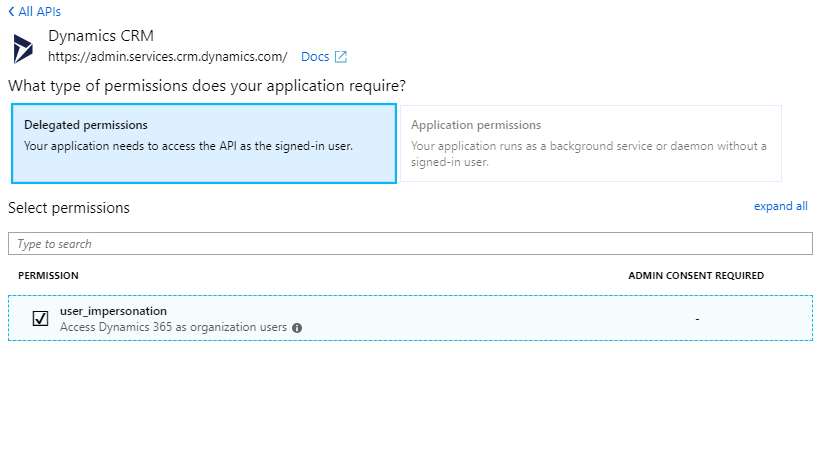
Next we need to add a secret key (Please copy and save key as you will not be able to see it once you move away from the page)
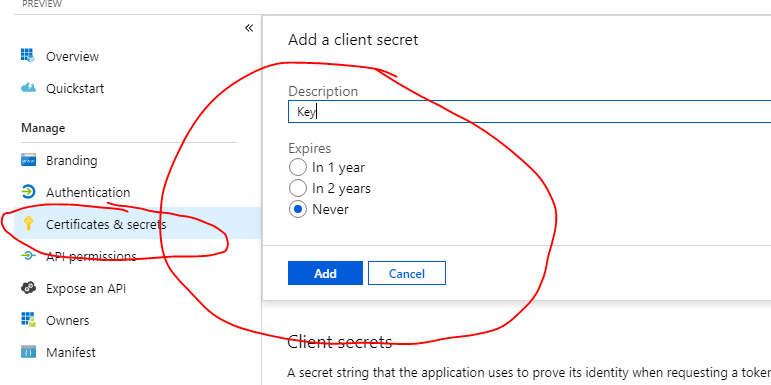
Next we need to copy Application ID (Client ID) (GUID) from Overview screen
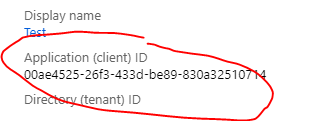
We are done with the Azure bit, now we need to move to Dynamics 365 and register an application user with App ID
Go to Settings>Security>Users and select Application Users view
then click on new

Give it a name, paste application ID from azure into Application ID field, fill in name and email
Save it
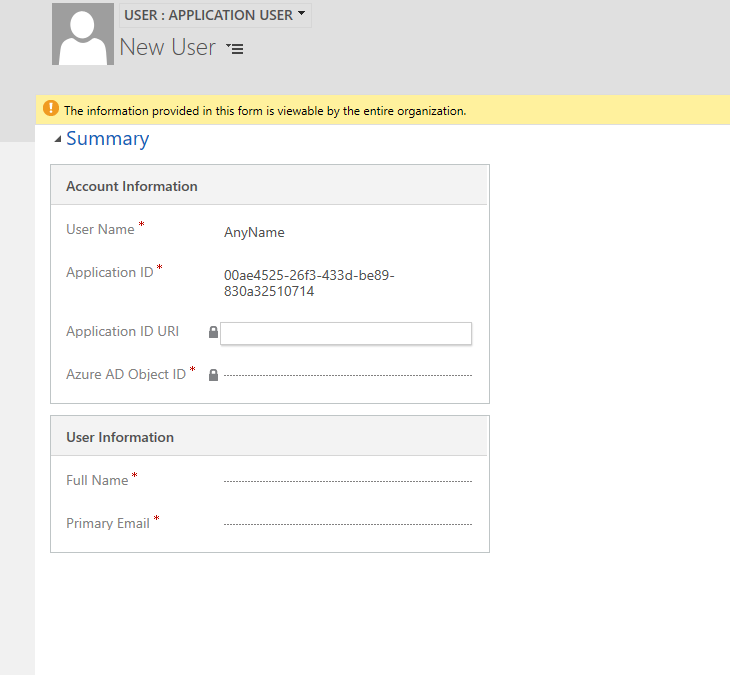
Now part 3 i.e. actual console application
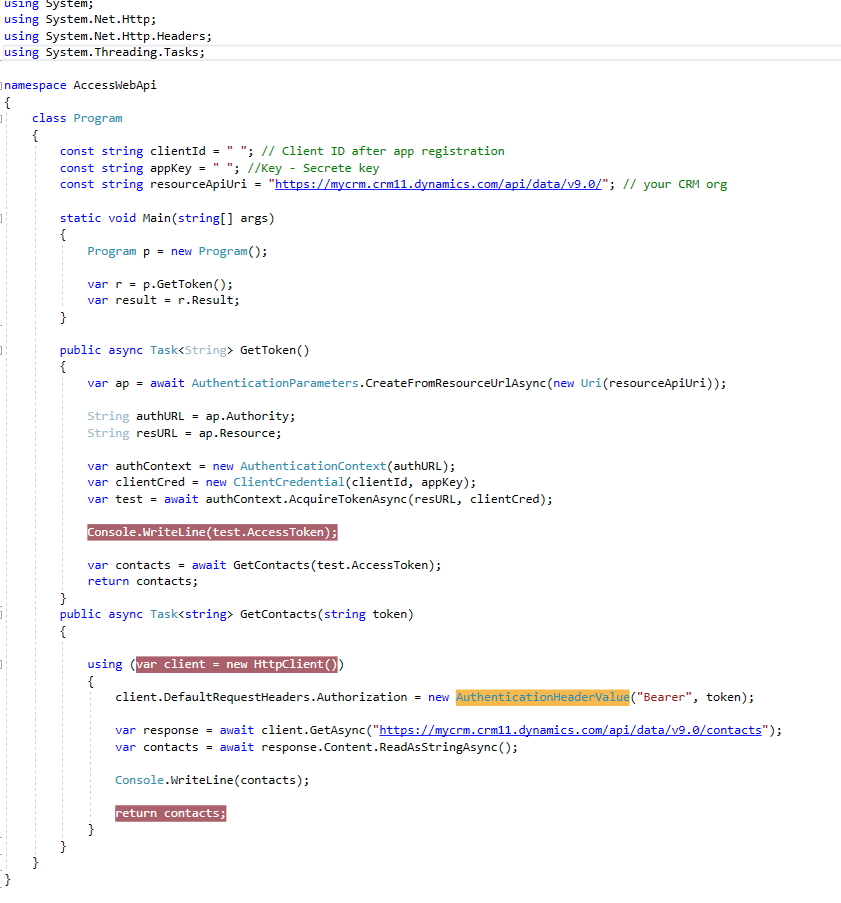
using Microsoft.IdentityModel.Clients.ActiveDirectory;
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Threading.Tasks;
namespace AccessWebApi
{
class Program
{
const string clientId = ” “; // Client ID after app registration
const string appKey = ” “; //Key – Secrete key
const string resourceApiUri = “https://mycrm.crm11.dynamics.com/api/data/v9.0/”; // your CRM org
static void Main(string[] args)
{
Program p = new Program();
var r = p.GetToken();
var result = r.Result;
}
public async Task GetToken()
{
var ap = await AuthenticationParameters.CreateFromResourceUrlAsync(new Uri(resourceApiUri));
String authURL = ap.Authority;
String resURL = ap.Resource;
var authContext = new AuthenticationContext(authURL);
var clientCred = new ClientCredential(clientId, appKey);
var test = await authContext.AcquireTokenAsync(resURL, clientCred);
Console.WriteLine(test.AccessToken);
var contacts = await GetContacts(test.AccessToken);
return contacts;
}
public async Task GetContacts(string token)
{
using (var client = new HttpClient())
{
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue(“Bearer”, token);
var response = await client.GetAsync(“https://mycrm.crm11.dynamics.com/api/data/v9.0/contacts”);
var contacts = await response.Content.ReadAsStringAsync();
Console.WriteLine(contacts);
return contacts;
}
}
}
}
In addition to above, you will need to install following nuget packages